Workflow Kit
The MatchPoint Workflow Kit is a functionality of MatchPoint that provides a mechanism for easy implementation of item-based workflows. All the functionality is based on the standard interfaces of the MatchPoint API, namely the MatchPoint framework mechanism, list event handlers, context menus and of course the tagging mechanism.
The feature set of a MatchPoint workflow is quite similar to a standard SharePoint workflow created with the SharePoint designer. However, there are some differences that are notable:
- Since a MatchPoint workflow utilizes the MatchPoint configuration mechanism, configuration is very easy and deployment to different systems requires only that the configuration file is copied to the MatchPoint configuration document library. The workflow can then be used on any SharePoint list that is enabled for MatchPoint tagging.
- Using the MatchPoint list event handler and (Data Grid) context menu, the functionality of the workflow integrates directly into SharePoint and the workflow is available on the list item, however it is accessed.
- The configuration file of a MatchPoint workflow allows the comfortable definition of forms that can be used to request user input for the workflow.
- A MatchPoint workflow allows more complex flows than a standard SharePoint workflow, e.g. loops.
- Within a workflow, all standard MatchPoint functionalities like the expression engine can be used, e.g. to automatically apply tags to the workflow item.
Terminology and Functionality
The workflow engine follows the concept of a state machine. Different triggers can be used to reach an initial state. Within a state, entry actions can be defined which are executed when an item is routed to that state.
Transitions between the states can be used to reach the next state. It is possible to provide user input fields on a UserTransition. These input values can be processed on the further workflow.
A running workflow is called a "workflow instance". MatchPoint can run multiple instances of the same workflow on a list item, if configured in the WorkflowConfiguration (EnableMultipleInstances).
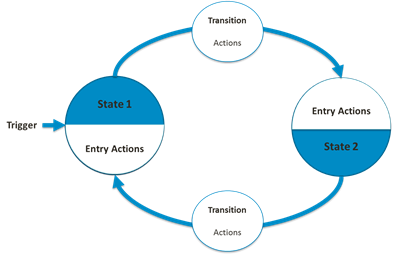
Activation
MatchPoint Workflow Kit can be activated from SharePoint UI using Site Settings -> Site Collection Features or by using the SharePoint Management Shell.
The feature receiver ensures the data grid context menu in the MatchPointConfiguration and creates a hidden workflow instance list on the site collection where the workflow engine persists information on running workflows, variable values and current workflow state.
- The Site Collection feature "MatchPoint Workflow Kit" must be activated
- The list on which the workflow should be used must be tagging enabled
- A WorkflowConfiguration must be created
- If you want to use UserTransitions / ContextMenuTriggers you need to have DataGrid with a TitleColumn and the setting "ShowCustomContextMenu" enabled
Workflow Kit Configuration Elements
States
A WorkflowState element represents a state of the workflow item. A state can have any number of EntryAction elements that are executed when the item reaches the state. Further a state can have any number of WorkflowTransition elements that are used to route the item to another state.
Triggers
Triggers define the entry point for a workflow. A trigger has a set of matching conditions that can be used to control trigger matching (e.g. UrlPrefix that has to match or several tags).
Workflows can be triggered in three different ways:
Trigger | Functionality |
---|---|
ContextMenuTrigger | A ContextMenuTrigger starts a workflow based on a user action, e.g. the user has to open the context menu from the item he wants to start the workflow an execute ("click") the workflow start action. One or more UserTransition elements can be defined. A context menu trigger works just like a user transition between two states. |
NewItemTrigger | A NewItemTrigger starts a workflow automatically when a new list item is created. |
UpdateItemTrigger | An UpdateItemTrigger starts a workflow automatically when a list item is updated. |
Transitions
A WorkflowTransition
element moves a workflow item from one state to
another. These transitions are triggered either automatically
(ConditionalTransition)
or by the user
(UserTransition)
from the workflow item's display form or from its context menu.
Conditional Transitions
Conditional transitions are based on a condition that is defined as a MatchPoint expression. If the condition evaluates to true, the transition is executed. A conditional transition performs a set of actions.
User Transitions
A user transition is a transition that is triggered by the user. It can be executed either from the title column or the link column context menu (option ShowCustomContextMenu enabled) in a data grid or from the item view form. Within a user transition, a user input form can be configured to provide MatchPoint user input fields to the user.
The following fields are available in the user transition form:
- AttachmentsField Class
- BooleanField Class
- ChoiceField Class
- CompositeField Class
- DateField Class
- ExpressionField Class
- ImageTagField Class
- LinkField Class
- NumberField Class
- PersonField Class
- RichTextField Class
- SectionField Class
- TagChoiceField Class
- TermChoiceField Class
Actions
Actions can be added as "EntryActions" for States or as Actions in UserTransitions and Conditional Transitions. The actions are the same as the MatchPoint Form Web Part uses, in the form of PostActions and BeforeUpdateActions. It's also possible to provide own actions if the BaseAction class is extended.
- CheckInFileAction Class
- CheckOutFileAction Class
- CopyFileAction Class
- CreateListItemAction Class
- CreateTaskAction Class
- CreateWebAction Class
- CreateWorkspaceAction Class
- DeleteFileAction Class
- DeleteItemAction Class
- EvaluateAction Class
- ForEachAction Class
- ModifyItemAction Class
- ModifyUpdatableAction Class
- ModifyWorkspaceAction Class
- SendMailAction Class
- SetFieldAction Class
- SetPermissionsAction Class
- SetTagsAction Class
- SetVariableAction Class
- SwitchAction Class
Workflow Tags
It may be useful to maintain the current workflow state of an item with a tag that is automatically applied. A TagDefinition element within the workflow configuration file can be used to specify a parent tag for the workflow tags as well as a tag provider. If such a tag definition is configured, a tag is automatically created for each state within the workflow and the tag corresponding to a workflow state is applied to the item.
Expression Variables and Extensions
Within a MatchPoint workflow, expressions are used to define the behavior of the workflow. To simplify access to the workflow instance and to the list item on which the workflow is running, the following additional expression variables were introduced:
Variable Name | Description |
---|---|
ListItem | Provides access to the list item (IUpdatable) on which the current workflow instance is executed. |
Transition | Provides access to the current transition within a user transition form. |
Updatable | Provides access to the updatable object (IUpdatable) on which the current workflow instance is executed. This is often the ListItem. |
Workflow | Provides access to the workflow configuration within a user transition form. |
WorkflowInstance | Represents the current instance of the workflow. Notably is the "Variables" property which allows access to variables within the workflow (values from a form within the workflow). |
The Workflowlist
As mentioned above, all information about a workflow instance is saved as a list item in the hidden workflow list which is created in the root web of the site collection in which the MatchPoint Workflow Kit feature is activated.
Some of the information stored in the list includes:
- The name and the config file name of the Workflow
- The current StateId
- The ID to the corresponding list item
- A JSON string with all variables of this workflow instance
That all variables are saved as a JSON string means that only types which can be serialized are supported as Workflow variables. Since all MatchPiont FormFields are serializable this is no problem. But if you want to store own variables, e.g. when passing a WorkflowVariableCollection to the Start method of the WorkflowConfiguration class, you have to make sure they are serializable.
It's also noteworthy, that this list is versioning enabled and therefore MatchPoint can easily display the workflow history (e.g. when opening the ContextMenu of a list item in a DataGrid and clicking "Show workflow history").
Accessing Workflows from the API
Starting a Workflow
There are two ways a workflow can be started programmatically, either client-side or server-side.
Server-Side (C#)
To start a MatchPoint workflow on a list item, the WorkflowConfiguration object of that workflow needs to be available. There are three ways to access the configuration object:
- Using the ConfigurationFile class:
- Call the constructor, using
the SPFile
object of the configuration file and the MPInstance object of
the MatchPoint installation.
- Use the LoadConfiguration(...) method to access the configuration object.
- Cast the IConfiguration object to a WorkflowConfiguration object.
- Using the WorkflowConfiguration class:
- Call the static method GetWorkflows(...), using
the MPInstance
object of the MatchPoint installation.
- Extract the workflow from the returned collection, i.e. filter by workflow name.
Once the WorkflowConfiguration object is available, the workflow can be started using the Start(...) method.
You can access available user transitions and available workflow states directly on the WorkflowConfiguration object.
In both cases, the SPListItem object of the item on which the workflow should be started is required. Optionally a dictionary of initial variables (which should be set on the workflow instance) can be specified. The Start(...) method will return a WorkflowInstance object which allows further access on the workflow.
Client-Side (JavaScript)
It is also possible to start a workflow from JavaScript (e.g within a composite web part). For this purpose the WorkflowManager.js provides the following method.
MP.WorkflowManager.StartWorkflow Function
MP.WorkflowManager.StartWorkflow(url, workflowId, transitionId, showForm)
The method has following parameters:
Url
: List item urlWorkflowId
: The guid of a workflow configuration fileTransitionId
: The guid of a workflow transitionShowForm
: Boolean parameter to control whether the user input form is shown or not.
The sample code below shows a call from a composite web part using a list data provider:
<a href="#" onclick="javascript:MP.WorkflowManager.StartWorkflow('{DataItem.Url}',
'2c708694-bab4-4c53-b0b8-3312ca20efc5',
'7daa2cab-b3f3-41bb-9bdd-75f01bd07deb',true)">Start Workflow</a>
Executing Transitions on a Workflow
C\
For executing user transitions on a running workflow, the SPListItem object of the item on which a workflow is running is required. MatchPoint registers an extension method GetWorkflowInstance(...) which returns the "WorkflowInstance" object.
On the WorkflowInstance object, the ExecuteUserTransition-method can be used. A Guid which identifies the user transition has to be specified. This ID can be determined manually from the workflow configuration file or programmatically by accessing the user transitions off the current workflow state:
The WorkflowInstance object provides a method named GetUserTransitions which returns all user transitions for the current workflow state.
The ExecuteUserTransitions(...)
method can only be called for user transitions that are available on the current workflow state.
JavaScript
The ExecuteUserTransition function on the WorkflowManager.js can be used to execute a user transition:
MP.WorkflowManager.ExecuteUserTransition(url, instanceId, transitionId, showForm);
The method has following parameters:
Url
: List item urlInstanceId
: The id of the workflow instanceTransitionId
: The guid of a workflow transitionShowForm
: Boolean parameter to control whether the user input form is shown or not.
On a list item, the GetWorkflowInstance()
extension method can be used
to retrieve the instance id.
<a href='#'
onclick='MP.WorkflowManager.ExecuteUserTransition("{DataItem.Url}",
"{DataItem.ListItem.GetWorkflowInstance().Id}",
"2e29c929-2e68-4129-ae20-090e0dbc9e90",
false)'>
Execute user transition
</a>